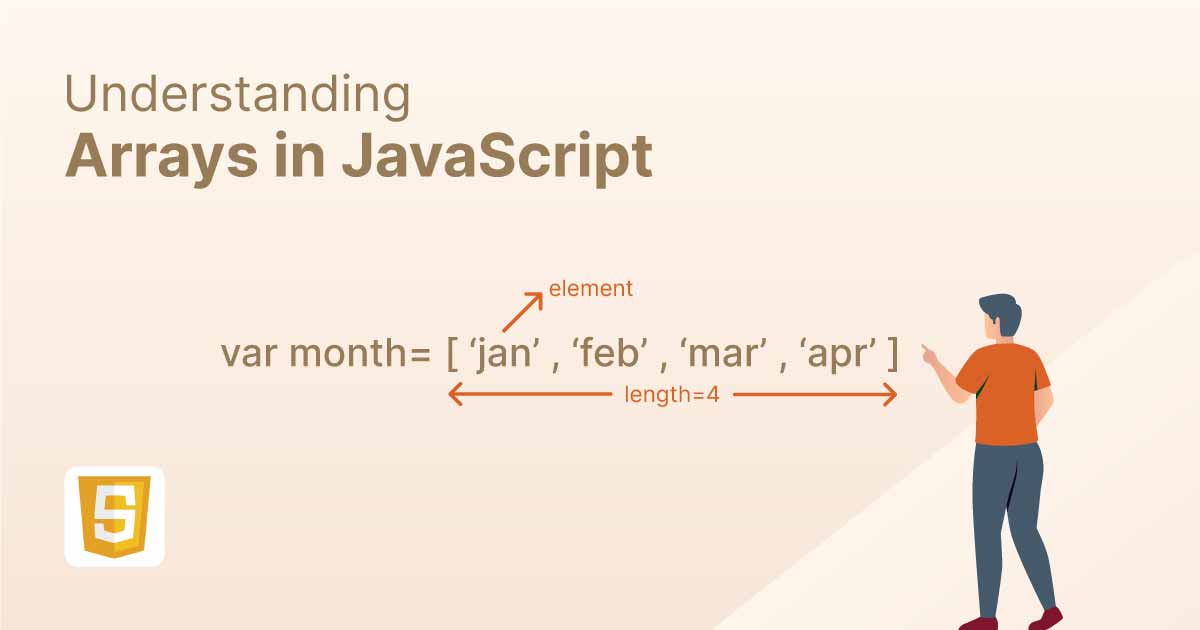
Understanding Arrays in JavaScript
Published Date: Aug. 29, 2024
An array in JavaScript is a special variable that can hold more than one value at a time. Arrays are a type of object and provide a way to store a collection of data in a single variable. They are particularly useful for storing lists, collections, or sets of related items.
Creating Arrays
There are multiple ways to create arrays in JavaScript:
1. Using Array Literals:
let fruits = ["Apple", "Banana", "Mango"];
2. Using the Array Constructor:
let fruits = new Array("Apple", "Banana", "Mango");
Accessing Array Elements
Array elements can be accessed using their index, which starts from 0.
let fruits = ["Apple", "Banana", "Mango"];
console.log(fruits[0]); // Output: Apple
console.log(fruits[1]); // Output: Banana
Modifying Arrays
You can modify array elements by assigning new values to existing indices.
let fruits = ["Apple", "Banana", "Mango"];
fruits[1] = "Orange";
console.log(fruits); // Output: ["Apple", "Orange", "Mango"]
Array Properties and Methods
- Length Property
let fruits = ["Apple", "Banana", "Mango"]; console.log(fruits.length); // Output: 3
- Push Method: Adds one or more elements to the end of an array.
let fruits = ["Apple", "Banana"]; fruits.push("Mango"); console.log(fruits); // Output: ["Apple", "Banana", "Mango"]
- Pop Method: Removes the last element from an array.
let fruits = ["Apple", "Banana", "Mango"]; fruits.pop(); console.log(fruits); // Output: ["Apple", "Banana"]
- Shift Method: Removes the first element from an array.
let fruits = ["Apple", "Banana", "Mango"]; fruits.shift(); console.log(fruits); // Output: ["Banana", "Mango"]
- Unshift Method: Adds one or more elements to the beginning of an array.
let fruits = ["Apple", "Banana"]; fruits.unshift("Mango"); console.log(fruits); // Output: ["Mango", "Apple", "Banana"]
- Concat Method: Merges two or more arrays.
let fruits = ["Apple", "Banana"]; let moreFruits = ["Mango", "Orange"]; let anotherFruits = ["Jackfruits", "Pineapple"]; let allFruits = fruits.concat(moreFruits, anotherFruits); console.log(allFruits); // Output: ["Apple", "Banana", "Mango", "Orange", "Jackfruits", "Pineapple"]
- Slice Method: Returns a shallow copy of a portion of an array.
let fruits = ["Apple", "Banana", "Mango", "Orange"]; let citrus = fruits.slice(2); console.log(citrus); // Output: ["Mango", "Orange"]
- Splice Method: Changes the contents of an array by removing, replacing, or adding elements.
let fruits = ["Apple", "Banana", "Mango"]; fruits.splice(1, 1, "Orange"); console.log(fruits); // Output: ["Apple", "Orange", "Mango"]
- forEach Method: Executes a provided function once for each array element.
let fruits = ["Apple", "Banana", "Mango"]; fruits.forEach(function(item, index) { console.log(index, item); }); // Output: // 0 Apple // 1 Banana // 2 Mango
- Map Method: Creates a new array with the results of calling a provided function on every element in the calling array.
let numbers = [1, 2, 3]; let doubled = numbers.map(function(number) { return number * 2; }); console.log(doubled); // Output: [2, 4, 6] // use function in Map function circleArea(radius) { return Math.floor(Math.PI * radius * radius); } let circles = [1, 2, 3, 4, 5]; // Example array of radii let areas = circles.map(circleArea); console.log(areas);
- Filter Method: Creates a new array with all elements that pass the test implemented by the provided function.
let numbers = [1, 2, 3, 4, 5]; let evenNumbers = numbers.filter(function(number) { return number % 2 === 0; }); console.log(evenNumbers); // Output: [2, 4]
- Reduce Method: Applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single value.
let numbers = [1, 2, 3, 4]; let sum = numbers.reduce(function(total, number) { return total + number; }, 0); console.log(sum); // Output: 10
Iterating Over Arrays
JavaScript provides several ways to iterate over arrays:
- for Loop:
let fruits = ["Apple", "Banana", "Mango"]; for (let i = 0; i < fruits.length; i++) { console.log(fruits[i]); }
- for...of Loop:
let fruits = ["Apple", "Banana", "Mango"]; for (let fruit of fruits) { console.log(fruit); }
- forEach Method:
let fruits = ["Apple", "Banana", "Mango"]; fruits.forEach(function(fruit) { console.log(fruit); });
Spread Operator
The ...
syntax is known as the spread operator in JavaScript. It allows an iterable (such as an array or a string) to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected, or an object expression to be expanded in places where zero or more key-value pairs (for object literals) are expected.
Examples and Uses of the Spread Operator
1. Copying an Array:
let originalArray = [1, 2, 3];
let copiedArray = [...originalArray];
console.log(copiedArray); // Output: [1, 2, 3]
2. Combining Arrays:
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
let combinedArray = [...array1, ...array2];
console.log(combinedArray); // Output: [1, 2, 3, 4, 5, 6]
3. Adding Elements to an Array:
let array = [1, 2, 3];
let newArray = [...array, 4, 5];
console.log(newArray); // Output: [1, 2, 3, 4, 5]
4. Spreading Elements in Function Calls:
function add(a, b, c) {
return a + b + c;
}
let numbers = [1, 2, 3];
console.log(add(...numbers)); // Output: 6
5. Copying Objects:
let obj1 = { a: 1, b: 2 };
let obj2 = { ...obj1 };
console.log(obj2); // Output: { a: 1, b: 2 }
6. Combining Objects:
let obj1 = { a: 1, b: 2 };
let obj2 = { c: 3, d: 4 };
let combinedObj = { ...obj1, ...obj2 };
console.log(combinedObj); // Output: { a: 1, b: 2, c: 3, d: 4 }