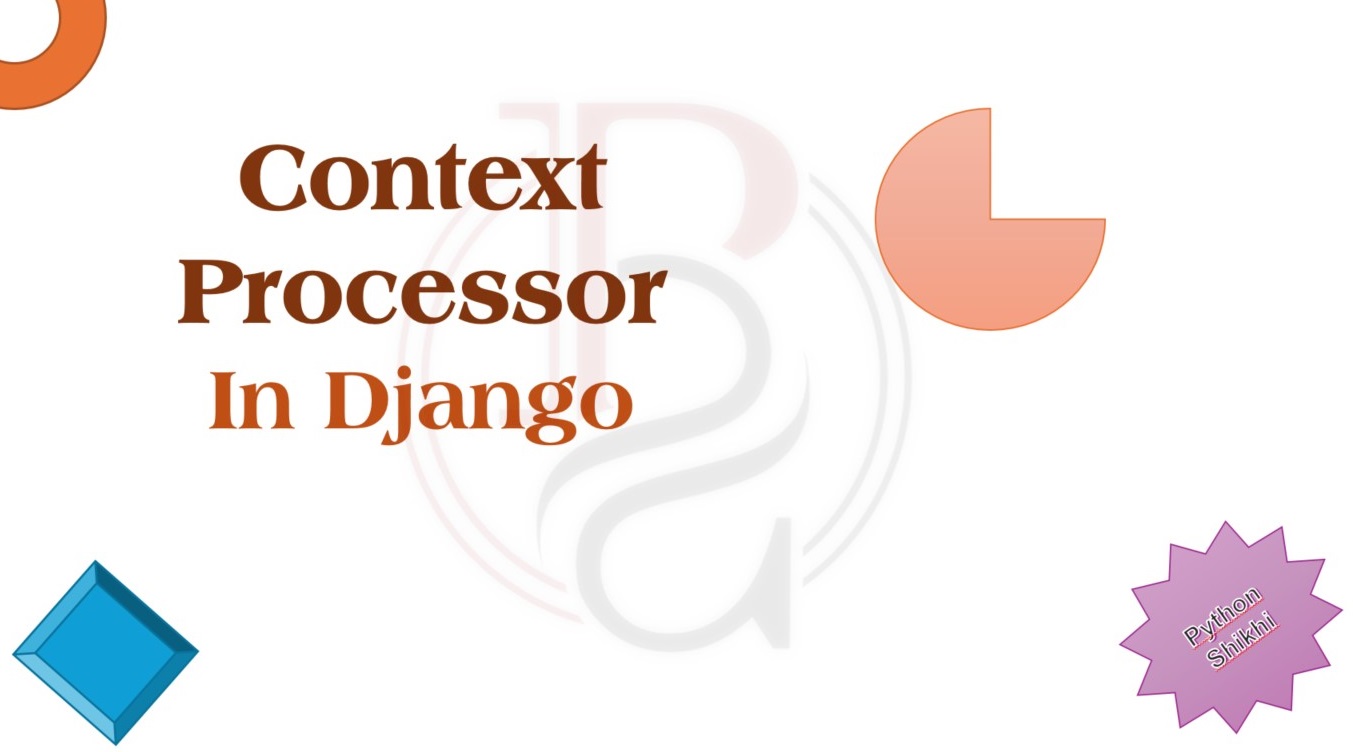
Introduction to Context Processor In Django Framework
Published Date: Sept. 20, 2024
1. What is a Context Processor?
In Django, a context processor is a function that takes an HttpRequest object as an argument and returns a dictionary of data that will be automatically included in the context of all templates.
For example, the active_page_processor function automatically adds the active_page variable to the context of every template, which helps identify which page is currently active.
2. Understanding active_page_processor.
The context processor function you mentioned:
def active_page_processor(request):
return {
'active_page': request.resolver_match.url_name
How it works
- request: This is the HTTP request object.
- request.resolver_match: This object contains information about the current URL pattern that is being matched.
- url_name: This is the name of the URL pattern that Django is using to serve the current page. If you’ve named your URLs in urls.py, this variable will hold that name.
This context processor automatically adds the current page's URL name to the template context, allowing you to use it in your HTML to highlight the active navigation link, for instance.
---
3. How to Use This Context Processor
Step-by-Step Usage:
1. Create the context processor function
In one of your app's directories, create a file called context_processors.py (if it doesn't exist already). Add the following function:
# your_app/context_processors.py
def active_page_processor(request):
return {
'active_page': request.resolver_match.url_name
}
2. Register the context processor in settings.py:
In the TEMPLATES setting of your settings.py, add this context processor to the context_processors list:
TEMPLATES = [
{
"BACKEND": "django.template.backends.django.DjangoTemplates",
'DIRS': [BASE_DIR,"templates"],
"APP_DIRS": True,
"OPTIONS": {
"context_processors": [
"django.template.context_processors.debug",
"django.template.context_processors.request",
"django.contrib.auth.context_processors.auth",
"django.contrib.messages.context_processors.messages",
"your_app.context_processors.active_page_processor", # Add this line
],
},
},
]
3. Use it in your template:
Now, in any template, you can use the active_page variable to determine the current page. For example, to highlight a navigation link:
<li>
<a href="{% url 'indexPage' %}" class="{% if active_page == 'indexPage' %}active{% endif %}">
Home
</a>
</li>
<li>
<a href="{% url 'aboutPage' %}" class="{% if active_page == 'aboutPage' %}active{% endif %}">
About
</a>
</li>
This will automatically add the active class to the link of the current page, helping with navigation styling.
4. Use Cases for Context Processors
Here are some common use cases for context processors:
1. Global Variables
If you have data that should be available across multiple views (or all views), you can use a context processor to make it globally accessible to all templates without needing to pass it through every view.
Example:
If you want to show site-wide settings like the site name or tagline, you can use a context processor to include this data globally in all templates.
def global_settings(request):
return {
'site_name': 'My Awesome Site',
'tagline': 'The best site on the web!',
}
2. User Information
You can use a context processor to make user-specific data available globally, such as showing a user's profile picture or notifications in the header of your website.
Example: Automatically include user profile information on all pages.
def user_profile(request):
if request.user.is_authenticated:
return {
'user_profile': request.user.profile, # Assuming you have a Profile model linked to the User model
}
return {}
3. Dynamic Menu/Navigation
As you did with active_page, you can use a context processor to dynamically highlight the active menu item or populate menus based on user permissions, roles, or other factors.
Example:
Provide a dynamic list of menu items:
def menu_items(request):
if request.user.is_authenticated:
return {'menu_items': ['home', 'dashboard', 'settings']}
return {'menu_items': ['home', 'login']}
4. Cart Information
In an e-commerce website, you may want to show the number of items in the shopping cart on every page. You can use a context processor for this.
Example:
def cart_info(request):
cart = request.session.get('cart', {})
return {
'cart_items_count': len(cart),
'cart_total_price': sum(item['price'] for item in cart.values())
}
5. Display User Notifications
If you have a notification system for your users, a context processor could load any unread notifications and make them available in your templates.
Conclusion
To summarize:
- A context processor allows you to add context variables to all your templates automatically.
- You can use them for things like active page tracking, global settings, user data, notifications, and more.
- The active_page_processor example you provided makes the current URL pattern name (url_name) available in templates, which is useful for things like highlighting the current page in navigation menus.
This is a very powerful tool in Django when you want to keep your views clean and DRY (Don’t Repeat Yourself), especially when you need to use the same context data across many templates.