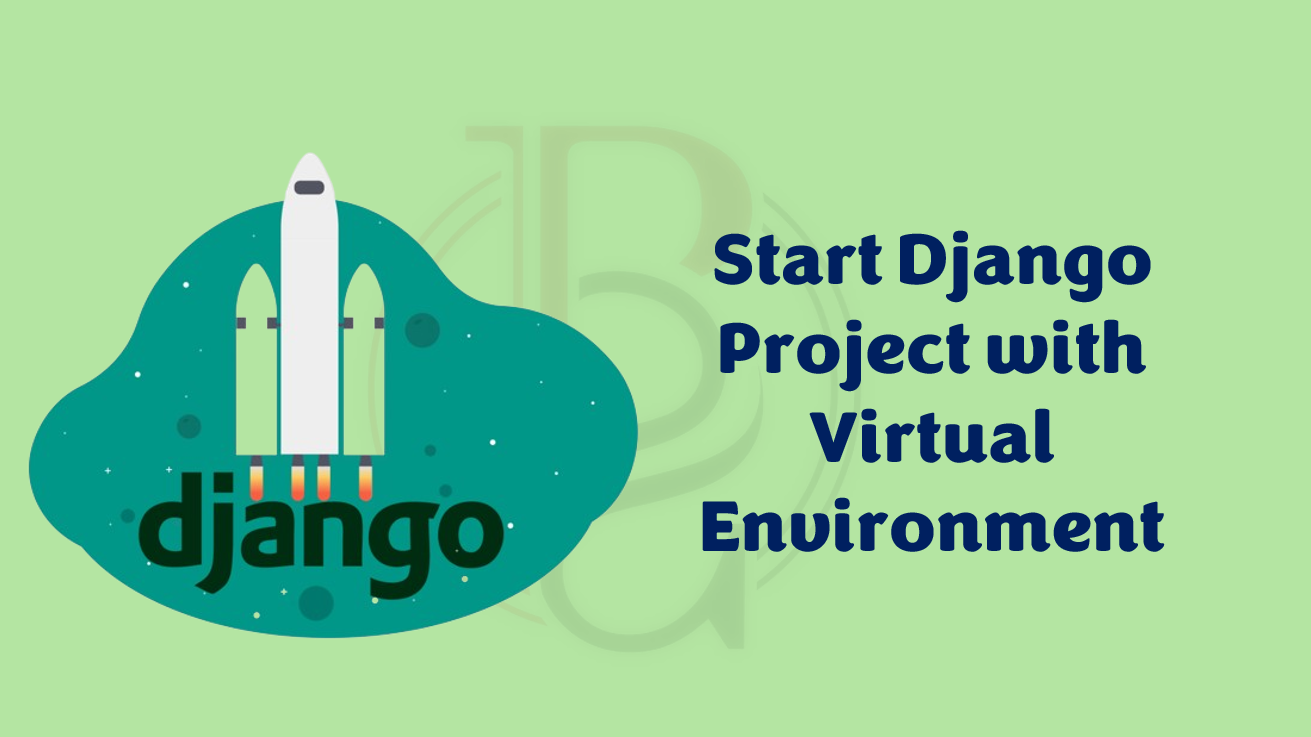
How to Start A django Project with Virtual Environment.
Published Date: Aug. 28, 2024
In today's session, we'll delve into starting a Django project with a virtual environment. By isolating dependencies, we ensure efficient and organized development. Learn how to set up Python, `virtualenv`, install Django, and initialize your project. Let's embark on this journey towards streamlined Django development.
A. Create Virtual Environment:
To create djnago project with virtual environment, we need to open a location/folder. After that we need to open that folder in vs code by using cmd in address bar. When a command prompt will open then we can create a virtual environment through command prompt. Below is the command line to create a virtual environment.
conda create -p venv python=3.8
In above command, 'venv' is the name of virtual environment and 3.8 is the python version.
After that create 'Y' to create virtual environment. It will take some time. After completing this step, virtual environment is been created already. Before activate desired virtual environment.
- Goto Terminal in vscode top menu bar.
- Select New Terminal.
- A New Terminal will open in bottom of the vscode. Then, click on + in right corner and select command prompt.
- If any base environment or other environment is running, first deactivate that environment. To deactivate it, write "deactivate" and click enter if not deactivate then developer should reach the environment by using cd/cd.. after that use "deactivate" command to deactivate the environment.
- By using cd/cd.. developer should reach the desired environment. To check the folder use 'dir' command. When developer observed that venv is present then s/he should use below command. Please use your folder path. Command is "conda activate 'folder_path' ". Example:
-
conda activate E:\Django\Python_Shikhi\Project_1\venv
As we use vscode so it is better to perform below command line in vscode command prompt.
B. Install Django:
By following command we can install django.
pip install Django
if any error come like "'pip' is not recognized as an internal or external command". Then upgrade the pip by using below command.
python -m ensurepip --upgrade
Then please check the version by using below command. if version will found then hopefully your pip will work correctly.
pip --version
C. Install mysql:
pip install mysql-client
D. Create Project:
To create project after install necessary module please write below command in console. In below command, i have created a project called myqrProject. Project Name you can keep as per your requirement.
django-admin startproject myqrProject
E. Settings File:
- Media file Path Settings:
import os # Build paths inside the project like this: BASE_DIR / 'subdir'. BASE_DIR = Path(__file__).resolve().parent.parent MEDIA_URL = '/media/' MEDIA_ROOT = os.path.join(BASE_DIR, 'media')
- Template Setting:
'DIRS': [BASE_DIR,"templates"],
- Database Settings:
DATABASES = { 'default': { 'ENGINE': 'django.db.backends.mysql', 'NAME': 'ps_codeblog1', 'USER': 'root', 'PASSWORD': 'Mahmud@100%', 'HOST': 'localhost', # or the hostname where your MySQL server is running 'PORT': '3306', # or the port on which your MySQL server is listening } }
- Static File Path:
STATICFILES_DIRS = [ BASE_DIR / "static", ]
F. Create New App: Below is the command line to start newapp.
python manage.py startapp app_name
After creating new app. It needs to listed up in INSTALLED_APPS in settings.py. Then, a urls.py file should create inside new_app folder. And below default setup should be write in urls.py.
from django.urls import path
from . import views
# please change accordingly.
urlpatterns = [
path('show_add_field', views.show_add_field, name='show_add_field'),
]
In above code, syntex is path('url_name',views.'function name', name='dynamic url name').
G. We need to add new_app urls in main project's urls.py
from django.urls import path, include
from django.conf import settings
from django.conf.urls.static import static
urlpatterns = [
path('admin/', include('app_name.urls')),
] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
H. Create 'templates' and 'static' folder under the main project.